Master Node.js
Backend Mastery Unfolded
|
Unlock the full potential of backend development with our "Master Node.js" course at Gopanear. Gain hands-on experience in building efficient, scalable server-side solutions, and elevate your programming skills to meet real-world challenges.
Register Now ₹9999/-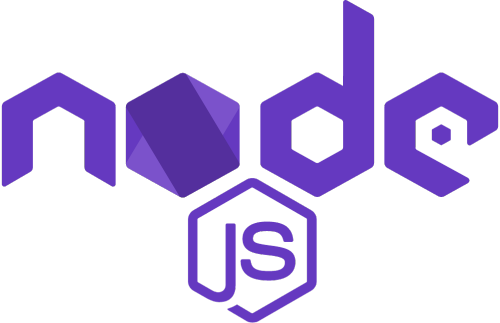
Comprehensive Curriculum
Course Coverage & Timeline
Embark on a 6-month journey through the intricacies of Node.js, dedicating one hour daily on weekdays. Our curriculum is designed to progressively build your skills through interactive videos, real-life projects, and consistent practice, culminating in proficiency in Node.js for backend excellence.
- Definition & Overview
- Definition & Overview
- Asynchronous and Event-Driven
- Single-Threaded with Scalability
- Cross-Platform Compatibility
- Examples of Node.js in action
- Common use-cases and success stories
- Examples of Node.js in action
- Common use-cases and success stories
- The V8 JavaScript Engine
- Non-blocking I/O Operations
- Event Loop and Callbacks
- Performance benefits
- Ecosystem and community support
- Versatility in web application development
- Node.js vs. Other Backend Technologies
- Installation and configuration
- Node.js and NPM (Node Package Manager)
- Creating a basic server
- Understanding modules and require syntax
- Core components of a Node.js application
- Best practices for organizing your project
- Concept of modules in Node.js
- Benefits of modular programming
- Writing custom modules
- Exporting and importing modules
- Overview of built-in core modules
- Utilizing npm to manage third-party modules
- Creating HTTP servers and clients
- Handling requests and responses
- Writing server-side code to handle HTTP requests
- Serving static files and HTML content
- Handling GET and POST requests
- Implementing routing and middleware logic
- Reading from and writing to files
- Working with file streams
- Understanding blocking and non-blocking operations
- Best practices for file system access
- Creating a file uploader
- Managing file directories
- Understanding URL components
- Using the URL module to parse URLs
- Retrieving and formatting query parameters
- Building query strings programmatically
- Using the URL module in HTTP servers
- Dynamic content based on URL parameters
- Overview of NPM and its role in Node.js development
- Setting up and configuring NPM
- Installing, updating, and uninstalling packages
- Understanding global vs. local package installation
- Semantic Versioning (SemVer) in NPM
- Using tags to specify package versions
- Managing project dependencies and devDependencies
- Resolving version conflicts and package updates
- Creating and publishing your own NPM packages
- Package naming conventions and best practices
- Understanding and mitigating package vulnerabilities
- Using tools like npm audit for package security
- Anatomy of the package.json file
- Mandatory fields and their significance
- Writing custom scripts for automation
- Predefined NPM script hooks
- Peer dependencies and bundledDependencies
- Configuring package.json for different environments
- Introduction
- How Node.js handles events
- Creating & emitting events
- Handling emitted events with listeners
- Real-world scenarios
- Error handling & event propagation
- Designing custom event emitters
- Observer patterns with event emitters
- Working with multiple listeners
- Managing event-driven state
- Memory management & event loop blocking
- Optimizing event emitter patterns
- Callback pattern & its usage
- Asynchronous operations with callbacks
- Introduction
- Refactoring callback-based code
- Managing errors in callbacks & Promises
- Techniques for controlling asynchronous flow
- Concept of streams
- Types of streams
- Handling stream events
- Buffer and stream interaction
- When and why to use streams
- Comparing streams with data handling methods
- Building a basic file upload server
- Handling multipart/form-data using middleware
- File uploads with streams for efficiency
- Managing file storage & upload errors
- Best practices for validating file uploads
- Security risks associated with file uploads
- Creating readable streams
- Handling stream data
- Implementing writable streams
- Piping data from readable to writable streams
- Using duplex & transform streams
- Error handling & backpressure management
- Overview
- The role of HTTP in web communication
- Setting up a server
- Handling routing & server responses
- Configuring server settings
- Best practices in server maintenance & security
- Parsing request methods
- Handling query parameters & request headers
- Setting response headers & status codes
- Sending response data & handling different content types
- Request handling with middleware
- Error handling & response optimization
- REST architecture and its principles
- Designing intuitive & efficient API endpoints
- CRUD operations
- Integrating database operations with APIs
- Authentication & authorization
- Testing APIs
- Overview of MySQL
- Benefits of using MySQL with Node.js
- Installing & configuring MySQL
- Establishing a connection with MySQL
- Designing tables & relationships
- Understanding primary and foreign keys, data types, and indexes
- Learning SQL syntax
- Managing database and tables
- Using MySQL modules in Node.js
- Writing & executing SQL queries
- MySQL connection pools
- Best practices
- CRUD operations with MySQL
- Asynchronous operations with Promises or Async/Await
- Complex SQL queries
- Optimizing queries for performance
- Building a sample application
- Real-world scenarios & error management
- Altering table structures
- Adding & modifying columns, indexes, and constraints
- Dropping databases & tables
- Recovery strategies
- Crafting SELECT statements
- Utilizing WHERE clauses
- INNER and OUTER joins
- Combining data from multiple tables
- Using aggregate functions
- GROUP BY and HAVING clauses
- ORDER BY clause
- Ascending & Descending sorting
- Conditions with AND, OR, and NOT operators
- Comparison operators & wildcards
- Pagination with OFFSET & LIMIT
- Subqueries
- IN, EXISTS, and BETWEEN operators
- Best practices in query optimization
- dentifying & resolving common performance issues
- WebSocket protocol
- WebSockets vs. HTTP
- Setting up Socket.io
- Sending & receiving real-time data
- WebSocket connections & events
- Designing the chat interface
- Server-client communication for chat features
- Adding user authentication & rooms
- Private messaging & group chats
- Scalability & performance
- Handling concurrency & data persistence
- Push notification mechanisms
- Setting up a push notification server
- Push notifications in web applications
- Handling notification delivery & user interactions
- Customizing notifications
- Analyzing & improving the push notifications
- Creating scalable & maintainable architecture
- Planning for data flow & component integration
- Structuring the project directory
- Best practices for code organization & modularity
- Step-by-step development of a full-stack application
- Implementing front-end, back-end, and database integration
- Writing tests for various components
- Debugging & fixing issues
- Preparing the application for deployment
- Choosing deployment platforms & services
- Monitoring & updating the application post-deployment
Learning Outcomes
Skills Gained
Master Node.js fundamentals and advanced topics over six months to build scalable, efficient backend systems. Equip yourself with master-skills for modern web applications.
Node.js Fundamentals
Understand the basics of Node.js, including its asynchronous programming model. Learn about Node.js architecture, event loops, and how it differs from traditional web servers.
Building RESTful APIs
Gain expertise in building RESTful APIs using Node.js. Learn to handle HTTP requests, route URLs to corresponding logic, and interact with databases for data retrieval and manipulation.
Working with Express.js
Master the use of Express.js, a popular Node.js framework. Learn to create robust server-side applications, set up middleware for request processing, and route handling.
Database Integration
Learn to integrate various databases like MongoDB and SQL with Node.js. Understand how to store, retrieve, and manipulate data effectively in a Node.js environment.
Real-Time Communication
Delve into building real-time web applications using Socket.io with Node.js. Learn about WebSocket protocol and how to implement real-time communication in your applications.
Authentication & Security
Understand the principles of securing Node.js applications. Learn about authentication, authorization, and best practices to protect sensitive data and prevent vulnerabilities.
Unit Testing & Debugging
Acquire skills in unit testing with frameworks like Mocha and Chai. Learn debugging techniques and error handling to maintain high-quality code.
Deployment & Performance Optimization
Learn the best practices for deploying Node.js applications and optimizing their performance. Understand server configuration, load balancing, and performance tuning for scalable applications.
Develop an in-depth understanding of Laravel's elegant syntax and its expressive, high-level abstraction of common web development patterns. Gain the ability to structure a robust backend for applications.
Learn to design, query, and manipulate databases with Eloquent ORM, mastering the art of handling data relationships and database migrations, ensuring data integrity and performance optimization.
Acquire the skills to craft scalable web applications from scratch, utilizing Laravel’s comprehensive features to create responsive, data-driven websites tailored to user needs.
Build RESTful APIs that seamlessly integrate with front-end applications, enabling fluid data exchange and expanding the functionality of your web applications across multiple platforms.
Embrace a robust test-driven development (TDD) approach, learning to systematically write and efficiently run automated tests with PHPUnit to ensure your code is reliable, maintainable, and of high quality.
Hone your debugging skills to swiftly identify and resolve issues, and optimize the performance of Laravel applications to handle high traffic and complex operations with ease.
Implement Laravel’s built-in security features to protect applications from common threats, understanding best practices for safeguarding user data and maintaining application integrity.
Apply your Laravel expertise to solve practical challenges, adapting the theoretical knowledge to create innovative solutions for real-world problems and user-centric applications.
MEET YOUR COACH
With over 10+ years of experience in web development and deployment, I have accumulated a wealth of knowledge and a treasure trove of industry insights. My journey through the years has been marked by significant contributions to numerous projects, which include everything from dynamic web applications to sophisticated, high-traffic websites.
- Versatile Developer
- Deployment Specialist
- Innovative Problem Solver
- Educator and Mentor
- Inspirational Approach
- Practical Wisdom
Join me in this exclusive webinar and gain the skills to confidently deploy your web projects, ensuring they perform seamlessly in the live environment. Secure your spot now and take the first step towards becoming a deployment expert.
MEET YOUR COACH
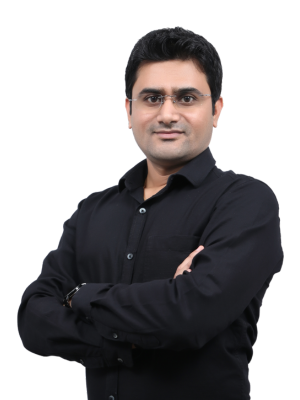
10+
years of experience
Sandip Gopani
CEO, Gopanear LLP
Interactive & In-depth
Webinar Format
Our goal is to make each webinar an interactive, informative, and engaging experience, ensuring you gain valuable knowledge and practical insights.
Live Sessions
Our primary mode of delivery is live webinars. Conducted in real-time, these sessions allow for up-to-date information and timely interaction. The live format ensures that content is dynamic, tailored to the audience, and responsive to the latest industry trends.
Live Coding and Demonstrations
Many of our webinars, especially those on technical subjects, include live coding sessions or live demonstrations. These practical segments allow you to see concepts in action and understand their real-world applications.
Q&A Sessions
Each webinar features a dedicated Q&A segment. Participants are encouraged to ask questions, providing an opportunity for in-depth discussion on specific topics or concerns.
Pre-Recorded Segments
Certain complex topics may be supplemented with pre-recorded segments. These are crafted to provide a clear and concise explanation of intricate concepts, ensuring you get a well-rounded understanding.
Polls and Surveys
Interactive polls and surveys are conducted during the webinar. These not only make the sessions more engaging but also help tailor the discussion to the audience’s interests and knowledge level.
Interactive Workbooks or Exercises
For certain topics, we provide interactive workbooks or exercises that you can work on during the webinar, enhancing your learning through practical application.
Enhance Your Node.js Skills with Expert-Led Training
Join our comprehensive program to cultivate in-demand backend development skills using Node.js.
Register Now ₹9999/-Transform your web development career with Node.js.
Embark on a journey to master backend development with Gopanear's "Master Node.js" course. Gain the expertise to build scalable and efficient web applications and APIs.Transform your development career with Node.js.
Essential Preparations
Technical Requirements
To ensure a smooth and effective learning experience in this webinars, participants will need to meet the following software & hardware requirements.
Web Browser
A modern and updated web browser such as Google Chrome, Mozilla Firefox, Safari, or Microsoft Edge. The browser should support HTML5 for optimal streaming of webinars.
Integrated Development Environment
For coding exercises, you'll need an IDE. We recommend Visual Studio Code, Sublime Text, or PHPStorm, especially if you're working with PHP and Laravel.
Git Version Control
Basic knowledge of Git for version control and accessing code repositories is recommended. Ensure Git is installed on your system.
Zoom Application
Most live sessions will be conducted via Zoom. Please download and install the Zoom client on your device. A basic (free) account is sufficient.
Headphones or Speakers
Good quality headphones or speakers for clear audio reception during webinars.
Note-taking Tools
Digital or physical note-taking tools for jotting down important points.
GET INFORMED
Answers to Your Questions
Gain clarity on the Node.js course structure, fees, and flexible learning paths. Our FAQs provide you with the knowledge to decide confidently on your tech journey.
Basic understanding of JavaScript and web development concepts is recommended before starting this course.
The course duration is 6 months, with a structured schedule to cover all topics comprehensively.
Yes, we emphasize practical learning with multiple hands-on projects to apply your Node.js skills in real-world scenarios.
Absolutely, creating RESTful APIs using Node.js is a key component of this course.
You'll attain a proficient level, capable of building and deploying scalable and efficient Node.js applications.
Yes, the course includes live sessions for deeper understanding and immediate query resolution.
Yes, we cover integrating MySQL with Node.js.
Assessments include quizzes, coding assignments, and a final project to evaluate your learning.
Yes, the course starts with basics and gradually advances, making it suitable for beginners.
We provide support through dedicated forums, email, and direct communication with instructors.
Absolutely, we continuously update the course to include the latest Node.js features and best practices.
Roles like Node.js Developer, Backend Developer, Full Stack Developer, and more will be suitable.
Group discounts are available. Please contact us for specific details and arrangements.
Yes, we cover key security practices for Node.js, including secure coding, authentication, and data protection techniques.
Yes, understanding asynchronous programming, including callbacks, promises, and async/await, is a fundamental part of the course.
Ready to take your development skills to the next level?
Join us today and unlock new possibilities in your career. The "Master Node.js" course at Gopanear provides an in-depth understanding of Node.js, covering everything from database integration to real-time communication.